Client-Server Architecture
The client-server model is a distributed application structure that partitions tasks between providers of a resource or service (servers) and service requesters (clients). This architecture is the foundation of most networked applications, from web browsers and email clients to database systems and online games.
Understanding the Client-Server Model
In the client-server model:
- Servers provide resources, data, services, or functionality to clients
- Clients request and use the resources, data, services, or functionality provided by servers
- Communication between clients and servers occurs over a network using a predefined protocol
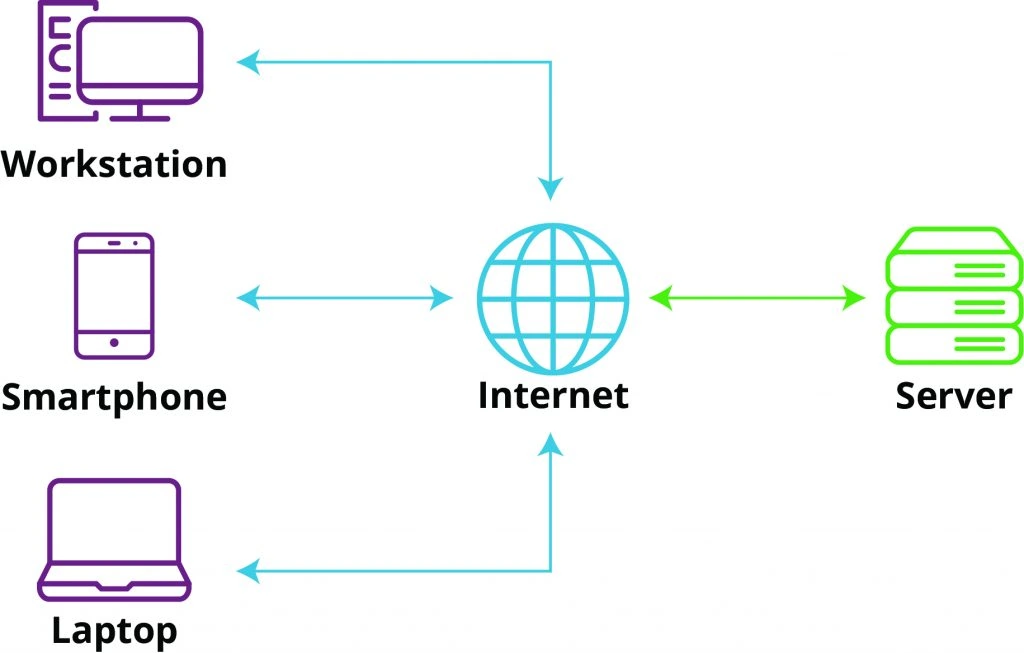
Key Characteristics
- Centralized Control: Servers centralize resources and control access to them
- Scalability: The model can scale by adding more servers or distributing load
- Separation of Concerns: Clients and servers have distinct roles and responsibilities
- Network-Based: Communication occurs over a network using protocols like TCP/IP
- Request-Response Pattern: Clients send requests, servers process them and send responses
Client-Server Communication Patterns
Request-Response
The most common pattern where a client sends a request and waits for a response from the server.
Example: HTTP web requests, database queries
Publish-Subscribe
Clients subscribe to specific types of messages, and servers publish messages to subscribers.
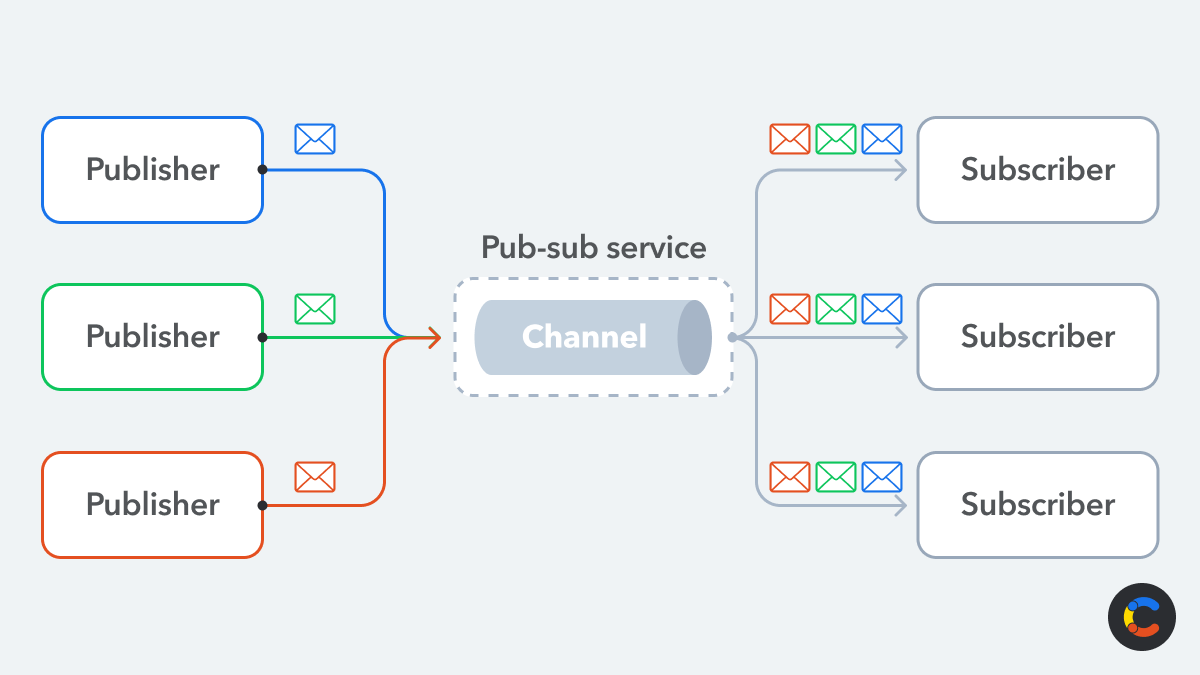
Example: Message queues, event notification systems
Push
Servers proactively send data to clients without explicit requests.
Example: Real-time notifications, streaming updates
Long Polling
Clients make a request and the server holds the connection open until new data is available.
Example: Chat applications, real-time dashboards
Designing a Client-Server Application
When designing a client-server application, consider the following aspects:
1. Protocol Design
Define how clients and servers communicate, including:
- Message Format: Structure of requests and responses (e.g., JSON, XML, binary)
- Message Types: Different kinds of messages (e.g., request, response, notification)
- Error Handling: How errors are reported and handled
- Versioning: How protocol changes are managed over time
Example of a simple text-based protocol:
Example of a binary protocol structure:
2. Connection Management
Decide how connections between clients and servers are established and maintained:
- Connection-Oriented vs. Connectionless: TCP vs. UDP
- Persistent vs. Non-Persistent: Keep connections open or close after each transaction
- Connection Pooling: Reuse connections to reduce overhead
- Heartbeats: Periodic messages to verify connection status
- Reconnection Strategy: How clients handle server disconnections
3. Concurrency Model
Determine how the server handles multiple clients simultaneously:
- Process per Client: Fork a new process for each client
- Thread per Client: Create a new thread for each client
- Thread Pool: Use a fixed number of threads to handle client requests
- Event-Driven: Use non-blocking I/O and event loops
- Asynchronous I/O: Use asynchronous operations to handle multiple clients
4. State Management
Define how application state is maintained:
- Stateless: Each request contains all necessary information
- Stateful: Server maintains client state between requests
- Session Management: How client sessions are tracked
- Persistence: How data is stored and retrieved
5. Security Considerations
Implement security measures to protect the application:
- Authentication: Verifying client identity
- Authorization: Controlling access to resources
- Encryption: Protecting data in transit
- Input Validation: Preventing injection attacks
- Rate Limiting: Preventing abuse
Implementing a Robust Client-Server Application
Let's implement a more robust client-server application that addresses some of the considerations mentioned above.
Protocol Definition
First, let's define a simple protocol for our application:
Server Implementation
Here's a more robust TCP server implementation:
Client Implementation
Here's a corresponding client implementation:
Best Practices for Client-Server Applications
Server-Side Best Practices
- Graceful Shutdown: Handle signals and clean up resources properly
- Resource Limits: Set limits on connections, memory usage, etc.
- Logging: Implement comprehensive logging for debugging and monitoring
- Error Handling: Handle all possible error conditions
- Security: Implement authentication, authorization, and encryption
- Scalability: Design for horizontal scaling
- Monitoring: Add health checks and performance metrics
Client-Side Best Practices
- Connection Management: Handle reconnection and failover
- Timeout Handling: Set appropriate timeouts for operations
- Error Recovery: Implement retry logic with backoff
- Resource Cleanup: Release resources when done
- User Feedback: Provide meaningful feedback about connection status
- Offline Mode: Consider implementing offline functionality when possible
Conclusion
The client-server architecture is the foundation of most networked applications. By understanding the principles and best practices of this model, you can design and implement robust, scalable, and secure networked applications.
In the next section, we'll explore socket options and configuration in more detail to optimize the performance and behavior of your socket-based applications.
Test Your Knowledge
Take a quiz to reinforce what you've learned
Exam Preparation
Access short and long answer questions for written exams